Smart contracts are a defining feature of blockchain technology, enabling it to go beyond being just a decentralized financial system or a trustless store of value. However, security remains a critical challenge that requires innovative solutions if blockchain is to achieve its full transformative potential.
At its core, smart contract security follows many of the same principles as traditional software security. It all starts with the code. Poorly written code that doesn’t follow best practices increases the attack surface, making it easier for malicious actors to exploit vulnerabilities.
This blog delves into the most critical best practices for developing smart contracts, providing technical explanations and actionable insights.
1. Understand the Basics of Solidity and Blockchain
Master the Language
- Learn Solidity: Solidity is the de facto language for Ethereum smart contracts, offering a robust yet nuanced environment for development. It is statically typed and supports inheritance, libraries, and user-defined types. Developers should thoroughly understand its syntax, features, and quirks to minimize bugs.
- Understand EVM Behavior: The Ethereum Virtual Machine (EVM) is the runtime environment for executing smart contracts. Knowledge of how the EVM handles bytecode, memory, and storage is essential for writing gas-optimized and secure contracts.
Learn Blockchain Principles
- Decentralization: Blockchain distributes data across a global network of nodes, ensuring redundancy and trustlessness. Developers must design contracts with this distribution in mind.
- Immutability: Once deployed, smart contracts cannot be altered. This immutability enforces trust but necessitates meticulous planning and testing during the development phase.
- Gas Costs: Gas is the measure of computational work required to execute operations on Ethereum. High gas costs can deter users, so optimizing for gas efficiency is critical.
- Transparency: Blockchain ensures that all transactions and data are recorded on a public ledger, allowing anyone to verify and audit activity without relying on a central authority.
- Permissionlessness: Operates without barriers, enabling anyone with internet access to participate, interact, and transact without needing approval from intermediaries or centralized entities.
2. Adopt Secure Coding Practices
Minimize Attack Surfaces
- Limit Contract Size: Large contracts are more prone to errors and vulnerabilities. Modular design, where functionality is divided across smaller, reusable contracts, improves both security and maintainability.
- Safeguard External Calls: External calls introduce unpredictability, as the called contract may have malicious intentions or unexpected behavior. Use the “checks-effects-interactions” pattern to handle external calls safely.
- Solidity Design Patterns: Solidity design patterns, like the Checks-Effects-Interactions, Access Control, Factory Pattern, Pull-over-Push Method, State Machine and Proxy Pattern, help minimize attack surfaces by structuring code to prevent common vulnerabilities like reentrancy and unauthorized access. Using these patterns ensures your smart contracts are more secure and resistant to exploitation.
Validate Input
- Input Sanitization: Every external input is a potential attack vector. Validate inputs rigorously to ensure they conform to expected formats and ranges. For example, verify addresses and ensure numeric inputs fall within acceptable bounds.
- Access Control: Implement strict access control mechanisms. Use Solidity’s access modifiers like onlyOwner or role-based access control libraries to restrict critical functionality to authorized entities.
Protect Against Common Vulnerabilities
- Reentrancy: Reentrancy attacks occur when an external contract is called before state changes are finalized. To mitigate this, always update the state before making external calls, and consider using mutexes or reentrancy guards.
- Integer Overflows/Underflows: Prior to Solidity 0.8.0, integer overflows and underflows were common pitfalls. Use SafeMath libraries in older versions or take advantage of Solidity’s built-in checked arithmetic in newer versions.
- Randomness: On-chain randomness is inherently insecure, as miners can manipulate block data. For critical operations requiring randomness, integrate off-chain solutions like Chainlink VRF (Verifiable Random Function).
3. Code Optimization and Gas Efficiency
Optimize Data Storage
- Use memory over storage: Storage operations are significantly more expensive than memory operations. Use the memory keyword for temporary variables to reduce gas costs.
- Packed Storage: Solidity organizes variables into storage slots. Align smaller variables of the same type to save storage space, reducing costs.
Function Optimizations
- Avoid Loops: Iterative operations within loops can quickly exceed block gas limits, leading to failed transactions. Minimize loops or replace them with more gas-efficient alternatives.
- Minimize State Changes: Writing to the blockchain is costly. Batch state updates or defer them when possible to optimize gas consumption.
Utilize Libraries and Inheritance
- Use Libraries: Libraries are a powerful way to encapsulate reusable logic. They can significantly reduce code duplication and help enforce modular design principles.
- Inherit Efficiently: Solidity supports contract inheritance, enabling developers to build upon existing functionality. Use inheritance judiciously to enhance code reuse while avoiding unnecessary complexity.
4. Testing and Auditing
Comprehensive Testing
- Unit Tests: Unit tests validate individual functions and ensure they perform as expected. Tools like Hardhat, Truffle, and Foundry provide robust frameworks for testing Solidity contracts.
- Integration Tests: Beyond unit tests, integration tests ensure that your contract interacts correctly with other components, such as external contracts or oracles.
- Edge Cases: Test for edge cases, such as maximum and minimum values, empty inputs, and unusual scenarios. Anticipate how your contract behaves under extreme conditions.
Audit Your Code
- Automated Analysis: Utilize static analysis tools like Slither, or Remix Analyzer to automatically detect vulnerabilities such as reentrancy, uninitialized variables, and race conditions.
- Manual Audits: Professional audits by experienced blockchain security firms provide a deeper review of your code. These audits are critical for high-value or complex contracts.
- Community Bug Bounties: Open your code to the community through bug bounty programs. Bug bounty platforms incentivize ethical hackers to find vulnerabilities before malicious actors do.
5. Follow Deployment Best Practices
Use Proxy Patterns
- Upgradeable Contracts: The immutability of smart contracts poses challenges when updates are required. Proxy patterns, such as OpenZeppelin’s TransparentUpgradeableProxy, decouple logic from storage, allowing contract upgrades without altering deployed addresses.
- Pause and Emergency Exit Functionality: Pause and Emergency Exit Functionality in smart contracts are safety mechanisms designed to protect users and funds during critical situations.
- Pause Functionality: Temporarily halts specific operations (e.g., transfers, withdrawals) to prevent further damage or exploitation. It is reversible, allowing operations to resume after resolving the issue.
- Emergency Exit Functionality: Enables users to withdraw their funds or exit the system safely, even when the contract is in a compromised or paused state. This ensures user protection during emergencies.
Verify Contracts
- Source Code Verification: Publishing your contract’s source code on platforms like Etherscan increases transparency and fosters trust within the community. Verified contracts are easier to audit and debug.
Monitor Post-Deployment
- Track Events: Emit events for significant actions within your contract. Events provide a structured way to monitor contract activity and facilitate debugging.
- Watch for Exploits: Stay vigilant after deployment. Regularly analyze transaction logs for anomalies or unauthorized access attempts.
6. Adhere to Industry Standards
Use Established Frameworks
- OpenZeppelin: OpenZeppelin provides a suite of battle-tested libraries and contracts, such as ERC-20 and ERC-721 implementations. Leveraging these frameworks reduces development time and enhances security.
- ERC Standards: Following Ethereum Request for Comments (ERC) standards ensures compatibility and interoperability. Common standards include ERC-20 for fungible tokens and ERC-721 for non-fungible tokens (NFTs).
Maintain Documentation
- Comprehensive Docs: Thorough documentation of your codebase improves maintainability and helps other developers understand your work. Include detailed descriptions of functions, parameters, and design decisions.
- README Files: A well-crafted README file should provide a high-level overview of your contract’s purpose, setup instructions, and key functionalities.
7. Embrace Continuous Learning
Stay Updated
- Version Changes: Solidity evolves rapidly, introducing new features, bug fixes, and optimizations. Keep abreast of version changes and adapt your contracts accordingly.
- Security Practices: Blockchain security is an arms race. Follow updates from reputable sources like ConsenSys Diligence and OpenZeppelin to stay ahead of emerging threats.
Participate in Communities
- Forums and Groups: Engage with Ethereum and Solidity communities on platforms like Ethereum Stack Exchange, Reddit, and Discord. Collaborate with peers to exchange knowledge and solve problems.
- Contribute to Open Source: Open-source contributions not only improve your skills but also establish your reputation in the blockchain ecosystem.
Conclusion
Developing secure, efficient, and maintainable smart contracts requires a deep understanding of blockchain principles, rigorous coding practices, and a commitment to continuous learning. By adhering to these best practices, you can build trust, enhance functionality, and reduce vulnerabilities in your decentralized applications. Whether you’re a novice or a seasoned developer, these guidelines provide a roadmap for achieving excellence in smart contract development.
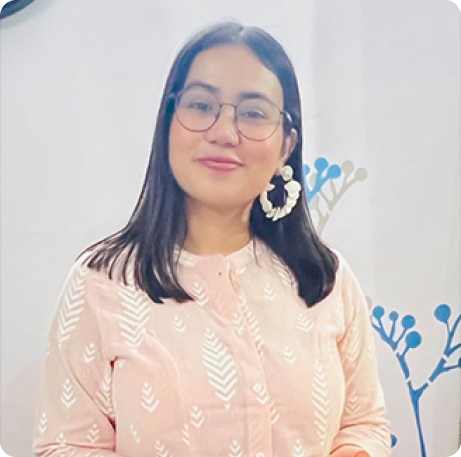